Ok, so this will likely be a long post, but here it is:
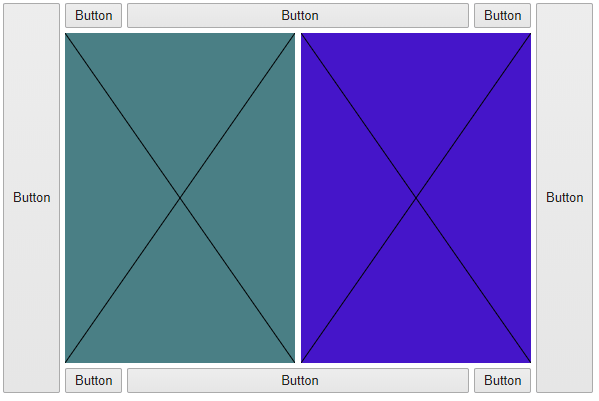
So, i basically create a silly fake lib, that in this case would represent an external js lib:
// this is a fake, external js lib for illustration purposes
function initLib(id) {
console.log("initLib: " + id);
}
function redrawCanvas(id, color) {
console.log("redrawCanvas: " + id);
var c = document.getElementById(id);
var cx = c.width;
var cy = c.height;
var ctx = c.getContext("2d");
ctx.fillStyle = color;
ctx.fillRect(0, 0, cx, cy);
ctx.beginPath();
ctx.moveTo(0, 0);
ctx.lineTo(cx, cy);
ctx.moveTo(cx, 0);
ctx.lineTo(0, cy);
ctx.stroke();
}
My .html looks like:
<body>
<canvas id="myCanvas1">
</canvas>
<script src="external_lib.js"></script>
<script src="Main.js"></script>
</body>
Ive then created a custom component that will either use, or create, a canvas and use the external lib to draw that canvas (obviously this could all be done in haxe code, but the idea is to show the custom component doing something “external”):
package custom;
import haxe.ui.containers.Box;
import js.Browser;
import js.Syntax;
import js.html.CanvasElement;
class MyExternalCanvas extends Box {
private var theCanvas:CanvasElement = null;
private var _col:String = null;
public var htmlId(null, set):String;
private function set_htmlId(value:String):String {
var el:CanvasElement = cast Browser.document.getElementById(value);
if (el == null) {
trace(value + " canvas not found, creating");
el = Browser.document.createCanvasElement();
el.id = value;
}
theCanvas = el;
this.element.appendChild(theCanvas); // will reparent if already exists
_col = "#" + StringTools.hex(Std.random(0xFFFFFF));
Syntax.code("initLib({0})", theCanvas.id); // should really create externs, im just using untyped js because its quick and dirty for an example
return value;
}
public override function validateComponentLayout():Bool {
var b = super.validateComponentLayout();
if (this.width > 0 && this.height > 0 && theCanvas != null) {
var usableSize = this.layout.usableSize;
theCanvas.width = Std.int(usableSize.width);
theCanvas.height = Std.int(usableSize.height);
Syntax.code("redrawCanvas({0}, {1})", theCanvas.id, _col); // should really create externs, im just using untyped js because its quick and dirty for an example
}
return b;
}
}
And thats it really, you can now use MyExternalCanvas
control anywhere in haxeui and is, for all intents and purposes a haxeui component. In my example, i also wanted to use it from xml, so i created a module.xml
on my classpath with these contents:
<module>
<components>
<class package="custom" />
</components>
</module>
Which will now expose any Component
derived controls to the xml. Which means now, i can define my layout as follows:
<hbox style="padding: 5px;" width="600" height="400">
<button text="Button" height="100%" onclick="this.width += 25" />
<vbox width="100%" height="100%">
<hbox width="100%">
<button text="Button" verticalAlign="top" />
<button text="Button" width="100%" onclick="this.height += 25" />
<button text="Button" verticalAlign="bottom" />
</hbox>
<hbox width="100%" height="100%">
<my-external-canvas id="ex1" htmlId="myCanvas1" width="50%" height="100%" />
<my-external-canvas id="ex2" htmlId="myCanvas2" width="50%" height="100%" />
</hbox>
<hbox width="100%">
<button text="Button" verticalAlign="top" />
<button text="Button" width="100%" onclick="this.height += 25" />
<button text="Button" verticalAlign="bottom" />
</hbox>
</vbox>
<button text="Button" height="100%" onclick="this.width += 25" />
</hbox>
Et Viola!
I hope that helps a little, using components this way is pretty useful and means they slide in nicely into haxeui.
Heres the full project: https://www.dropbox.com/s/cxucpy3dv7cfjg5/haxeui-core-custom_external_component.zip?dl=0
Cheers,
Ian